Friday, Feb 1, 2013
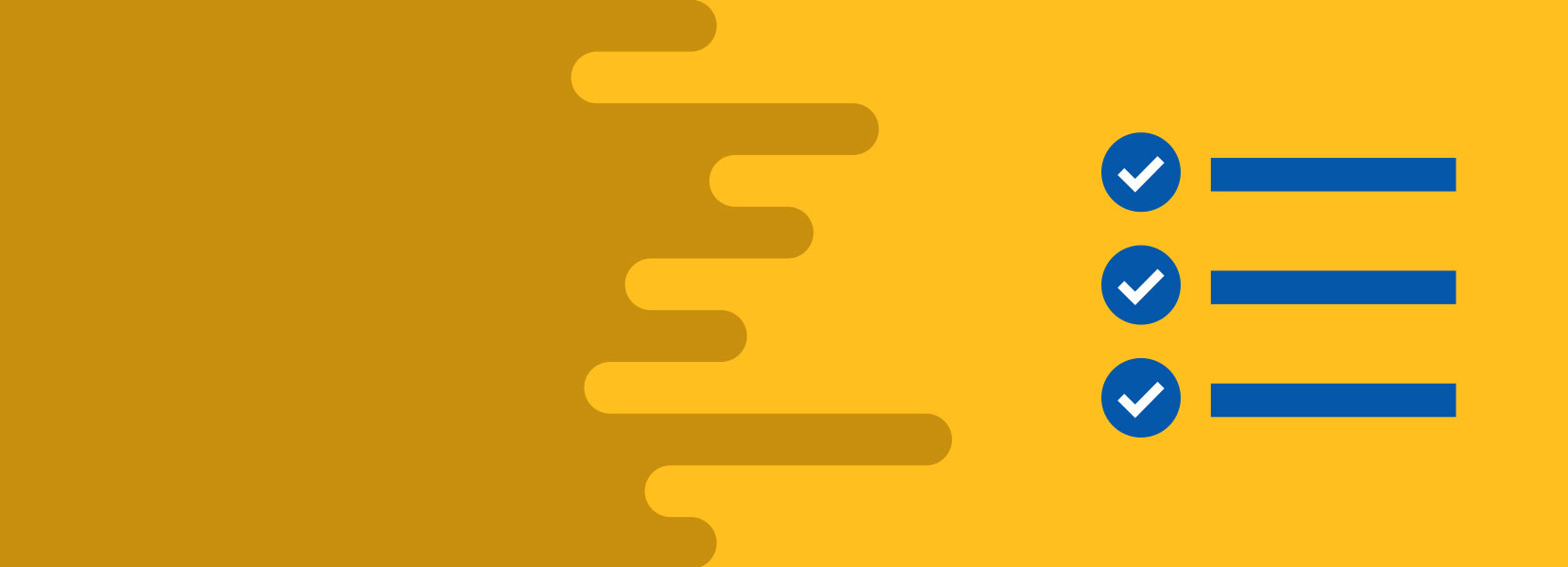
Learn how to use and customize the ListView control in Xamarin.Forms
When you start learning about Xamarin.Forms, sooner or later you’ll have to use ListView
as the control for displaying data, and because of that, it is one of the most popular controls in the Xamarin ecosystem.
Project setup
Since Visual Studio 2017 has many improvements compared to Visual Studio 2015, we’ll be using that version for this tutorial (read more here).
Open your Visual Studio instance, click File -> New -> Project, and under Templates navigation, select Cross-platform and then Cross Platform App. For this tutorial, I’ll name my project ListViewDemoApp. Once you select a name for your project, click Ok.
After that, another window will open - select Xamarin.Forms as UI Technology and PCL as a Code Sharing Strategy and click Ok.
Now we can start developing the application.
Creating and displaying data
The main goal here is to show you the most common scenario when working with ListView
control - binding the control with the collection of the data and adjusting the control to show that data in an appealing way. As a first step, we are going to create a class Player
which contains information about basketball players such as name, position, and team.
public class Player
{
public string Name { get; set; }
public string Position { get; set; }
public string Team { get; set; }
}
Now we need to create a collection of data that will be displayed in the ListView
control:
public static class PlayersFactory
{
public static IList<Player> Players { get; set; }
static PlayersFactory()
{
Players = new ObservableCollection<Player>() {
new Player
{
Name = "Kobe Bryant",
Position = "Shooting guard",
Team = "Los Angeles Lakers"
},
new Player
{
Name = "LeBron James",
Position = "Power forward",
Team = "Cleveland Cavaliers"
}
Notice that we are using ObservableCollection
here - it is used in case you want to add or remove an item from your collection and that change to be reflected on your ListView
control; then you must use it as a source of the data collection.
The next step is to bind the data collection to ItemSource
property of the ListView
:
playerListView.ItemsSource = PlayersFactory.Players;
We are done with the code behind at the moment, so we can focus on XAML and how we’d like to display the data:
<ListView HasUnevenRows="True" BackgroundColor="White" x:Name="playerListView">
<ListView.Header>
<ContentView Padding="0,5" BackgroundColor="White">
<Label FontSize="Large" TextColor="BlueViolet"
Text="NBA Players" HorizontalTextAlignment="Center" VerticalTextAlignment="Center" />
</ContentView>
</ListView.Header>
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition />
<ColumnDefinition />
</Grid.ColumnDefinitions>
<StackLayout VerticalOptions="FillAndExpand" Padding="5" Grid.Column="0">
<Label FontSize="17" Text="{Binding Name}" />
<Label FontSize="12" Text="{Binding Team, StringFormat='Team: {0}'}" />
</StackLayout>
<StackLayout VerticalOptions="End" Padding="5" Grid.Column="1">
<Label FontSize="12" Text="{Binding Position, StringFormat='Position: {0}'}" />
</StackLayout>
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
Removing items
Besides displaying, ListView
control also has the option for removing certain item from the collection. To have this feature, we need to define ContextAction
within ViewCell
node.
<ViewCell.ContextActions>
<MenuItem Clicked="OnDelete" CommandParameter="{Binding .}" Text="Delete" IsDestructive="True" />
</ViewCell.ContextActions>
Once a user clicks the Delete button in the upper right corner, the OnDelete
function will be triggered and Player
object will be passed to that function. The logic for removing item is defined in the MainPage.Xaml.cs
file:
private void OnDelete(object sender, System.EventArgs e)
{
var listItem = ((MenuItem)sender);
var player = (Player)listItem.CommandParameter;
PlayersFactory.Players.Remove(player);
}
The only thing we need to do now is to run the application and see how ListView
is looking.
You can download the complete code sample here. As you have seen, the control itself is pretty straightforward and does not require advanced knowledge of C# and XAML.
I recommend not to stop with this post, but get more details about the implementations of the ListView
control here.