Friday, Dec 29, 2017
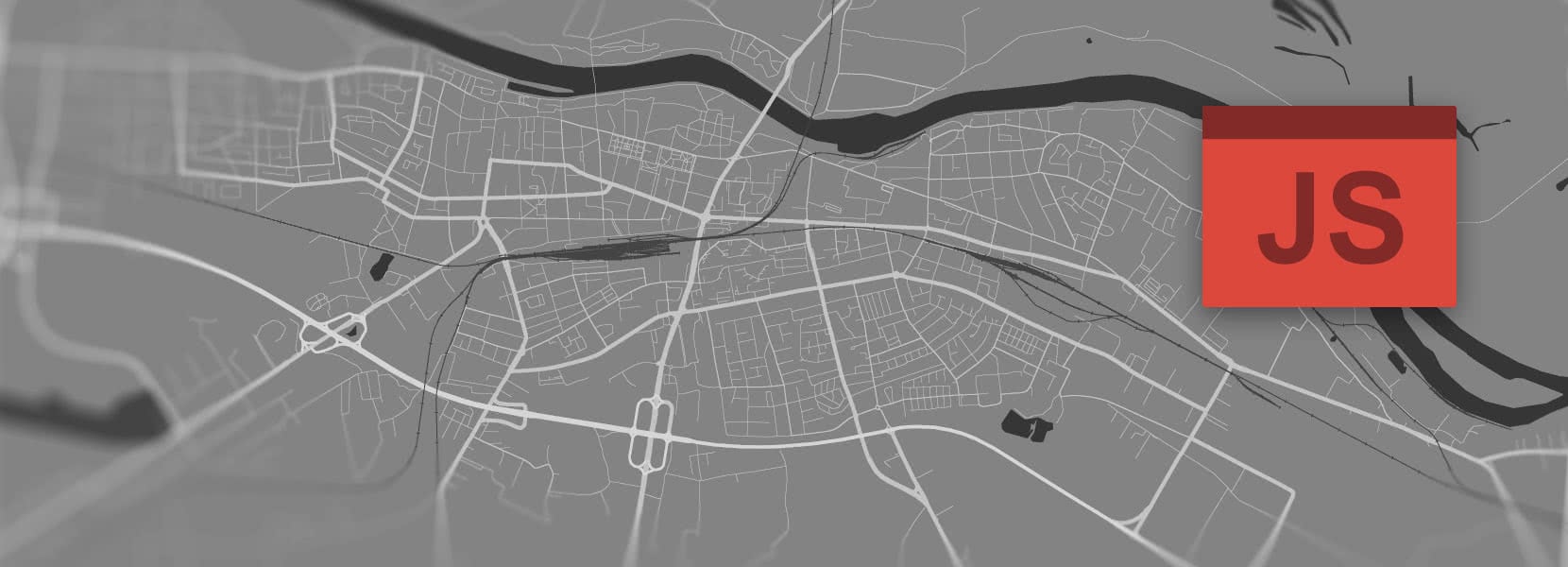
Google Maps JavaScript API - Intro
This article will teach you how to use Google Maps JavaScript API, and will cover initialization, custom styling, drawing basic shapes, adding markers, info window, and using geocoding service.
Map setup
First, we need the API key (we can get one from Google API Console):
- Create Google account if you don’t have one.
- Create a new project.
- Enable Google Maps JavaScript API.
- Under credentials, create new API key (you can restrict key only to your site).
Now that we have the key, we can add API script to the page. The best place to put it is just before closing the body
tag.
<script async defer src="https://maps.googleapis.com/maps/api/js?key=[YOUR KEY]&callback=initMap"></script>
To place a map, we need a div
, and its size can be defined by CSS. The next step will be to create JavaScript function initMap
, defined in script callback. This function will be called when JavaScript API is loaded and ready. Inside the function, we will create our map, and place it in the div
. To position the map, we will use options center
and zoom
, and to remove default buttons (Map, Satellite, Zoom in, Zoom out, and Street view), the option disableDefaultUI
is set.
We can create custom map styling using this tool, and then set styles
option on the map.
<div id="map" style="width: 640px; height: 400px;"></div>
var map;
function initMap() {
map = new google.maps.Map(document.getElementById("map"), {
center: {lat: 45.5518, lng: 18.6914},
zoom: 14,
disableDefaultUI: true,
styles: mapStyles
});
}
Now we have a styled and positioned map.
Drawing on the map
Let’s dive deeper and draw a red area on the map. We’ll do that by creating a new Polygon object. To set paths, we are passing an array of object which contains lat
and lng
properties. Next, we need to set fill
and stroke
options for polygon, and then we can add it to the map.
area = new google.maps.Polygon({
paths: areaCoordinates,
strokeColor: "#FF0000",
strokeOpacity: 0.6,
strokeWeight: 2,
fillColor: "#FF0000",
fillOpacity: 0.25
});
area.setMap(map);
Now, let’s draw a blue circle to demonstrate events when the area is clicked. We can make elements draggable, and we will do that for a circle. To show a couple of more events, we will disable moving blue circle from the red area by adding two more events. First, on dragstart
event, we will store current circle position. Then on dragend
event, we will check if the circle is inside the area (there is a handy function for that in API, google.maps.geometry.poly.containsLocation
). If the circle is not inside the area, we will move it back to the position that it was on when the dragstart
event happened, using the function setCenter
.
google.maps.event.addListener(area, "click", function(event) {
if(!circle){
addCircle(event.latLng);
}
});
function addCircle(center){
circle = new google.maps.Circle({
strokeColor: "#0000FF",
strokeOpacity: 0.8,
strokeWeight: 2,
fillColor: "#0000FF",
fillOpacity: 0.35,
map: map,
center: center,
radius: 300,
draggable: true,
zindex: 100
});
circle.addListener("dragstart", function() {
currentPosition = circle.getCenter();
});
circle.addListener("dragend", function() {
var insideArea = google.maps.geometry.poly.containsLocation(circle.getCenter(), area);
if(!insideArea) {
circle.setCenter(currentPosition);
}
});
}
Of course, there are other basic shapes, like polylines or rectangle.
Adding markers
And now to the main part. Let’s say we have a few locations we want to mark on the map, and some information to display for each location. To achieve that, we will use markers and info window.
When adding markers to the map, we can set the animation property using google.maps.Animation.DROP
animation. To “drop” markers one by one, we will use window.setTimeout
function, and increase the timeout for each subsequent marker.
To change the icon of the marker when hovering over it, we will use mouseover
and mouseout
events. You can also create your custom marker icons.
In this example, we will create only one info window. On marker click
event, we will set the content and open an info window.
infowindow = new google.maps.InfoWindow();
function addMarkers(){
for (var i = 0; i < data.length; i++) {
addMarkerWithTimeout(data[i], i * 200)
}
}
function addMarkerWithTimeout(data, timeout){
window.setTimeout(function() {
var marker = new google.maps.Marker({
position: data.position,
map: map,
animation: google.maps.Animation.DROP
});
google.maps.event.addListener(marker, "mouseover", function(event) {
marker.setIcon("http://maps.google.com/mapfiles/marker_green.png");
});
google.maps.event.addListener(marker, "mouseout", function(event) {
marker.setIcon();
});
marker.addListener("click", function() {
infowindow.setContent("<div><h2>" + data.value.h2 + "</h2><p>" + data.value.text + "</p></div>")
infowindow.open(map, marker);
});
markers.push(marker);
}, timeout);
}
Geolocation
If we only have the address of the location we want to use, either for positioning the map or the marker, we can use Geocoding Service
to get the position. To use it, we need to enable it in Google API Console. Here is how to do it:
geocodingService = new google.maps.Geocoder();
geocodingService.geocode( { "address": address}, function(results, status) {
if (status == "OK") {
var location = results[0].geometry.location;
alert("Lat: " + location.lat() + "\n" + "Lng:" + location.lng());
} else {
alert("Geocode was not successful for the following reason: " + status);
}
});
Another useful service that we’re only going to mention is DirectionsService
.
Live example
Below is not an image, but a map from our example. You can interact with it, zoom in and out, and change position using a mouse. You can click in the red area to add a circle, and the circle is draggable only inside that area. You can also try Geocoding service using the text input field and Coordinates button. To add markers, press the Add markers button, and to see the info window in action, select any marker.
You can get a complete code sample here.
To learn more, see the reference page.